Cron Expression Generator & Explainer - Quartz. Generate a quartz cron expression with an easy to use online interface. Convert a cron expression into a readable text that clearly explains when it will execute, and visualize the next execution dates of your cron expression. An expression without variables, such as 3 1 4, is called a numerical expression. An expression with variables, such as 5b2, is called an algebraic expression. Greatest common factor (GCF) the greatest factor two or more numbers have in common. Term a number, a variable, or a product of. Prepare 1.3: Expressions. Write a program to convert Fahrenheit to Celsius. This program will prompt the user for the Fahrenheit number and convert it to Celsius. The equation is: C = 5/9(F – 32) The program will prompt the user for the temperature, compute the Celsius value, and display the results. Evaluating expressions with one variable Our mission is to provide a free, world-class education to anyone, anywhere. Khan Academy is a 501(c)(3) nonprofit organization.
- 1 2+1 3+1 4 Fraction
- 1 3 Of 1 12
- 1 3+1 3+1 3 Equals
- Expressions 1 3 1 X 2
- 3.1 Simplify Rational Expressions
This section under major construction.
Regular expressions.
NFA.java,DFS.java,Digraph.java, andGREP.java.Running time.
M = length of expression, N = length of input.Regular expression matching algorithm can create NFA in O(M) timeand simulate input in O(MN) time.Library implementations.
Validate.java.
1 2+1 3+1 4 Fraction
Most library implementations of regular expressions use a backtrackingalgorithm that can take an exponential amount of time on someinputs. Such inputs can be surprisingly simple.For example, to determine whether a string of length N is matchedby the regular expression (a|aa)*b can take an amount of time exponentialin N if the string is chosen carefully. The table below illustratesjust how spectacularly that the Java 1.4.2 regular expression can fail.
The above examplesare artificial, but they illustrate an alarming defectin most regular expression libraries. Bad inputs do occur in practice.According toCrosbyand Wallach,the following regular expression appears in a versionof SpamAssassin, a powerful spam filtering program.
It attempts to match certain email addresses, but it takes an exponential time to match some strings in manyregular expression libraries including Sun's Java 1.4.2.
This is especially significant because a spammer could use a pathological return emailaddresses to denial-of-service attack a mail server that has SpamAssassin running.This particular pattern is now fixed because Perl 5 regular expresssionsuse an internal cache to short-circuit repeated matches at thesame location during backtracking.
These deficiencies are not limited to Java's implementation.For example, GNU regex-0.12 takes exponential time for matchingstrings of the form aaaaaaaaaaaaaac with the regular expression (a*)*|b*.Sun's Java 1.4.2 is equally susceptible to this one.Moreover, Java and Perl regular expressions support back references -the regular expression pattern matching problem for such extended regular expressions is NP hard,so this exponential blowup appears to be inherent on some inputs.
Here's one I actually wrote to try to find the last word before the string NYSE: regexp = '([ws]+).*NYSE';
Reference:Regular Expression Matching Can Be Simple And Fast (but is slow in Java, Perl, PHP, Python, Ruby, ..).Compares Thompson NFA with backtracking approach. Contains some performance optimizationsfor Thompson NFA. Also some historical notes and references.
Q + A
Q. Documentation on Java regular expression libraries?
A.Here is Oracle's guide to using regular expressions. It includesmany more operationsthat we will not explore.Also see the String methods matches(), split(), andreplaceAll(). These are shorthands for using the Pattern and Matcher classes.Here's some commonregular expression patterns.
Q. Industrial grade regular expressions foremail addresses, Java identifiers, integers, decimal, etc.?
A.Here's a library of useful regular expressionsthat offers industrial grade patterns foremail addresses, URLs, numbers, dates, and times.Try this regular expression tool.
Q.I'm confused why does (a | b)* match all strings of a's and b's,instead of only string with all a's or string with all b's?
A.The * operator replicates the regular expression (and not a fixed string that matches the regular expression). So the aboveis equivalent to ε | (a|b) | (a|b)(a|b) | (a|b)(a|b)(a|b) | ..
Q.History?
1 3 Of 1 12
A.In the 1940s, Warren McCulloch and Walter Pitts modeled neurons as finite automatato describe the nervous system.In 1956, Steve Kleene invented a mathematical abstraction calledregular sets to describe these models.Representation of events in nerve nets and finite automata.in Automata Studies, 3-42, Princeton University Press, Princeton, New Jersey 1956.
Q. Any tools for visualizing regular expressions?
A. Try Debuggerx.
Exercises
- Write a regular expression for each of the following sets of binary strings. Use only the basic operations.
- 0 or 11 or 101
- only 0s
Answers: 0 | 11 | 101, 0*
- Write a regular expression for each of the following sets of binary strings. Use only the basic operations.
- all binary strings
- all binary strings except empty string
- begins with 1, ends with 1
- ends with 00
- contains at least three 1s
Answers: (0|1)*, (0|1)(0|1)*, 1 | 1(0|1)*1, (0|1)*00,(0|1)*1(0|1)*1(0|1)*1(0|1)* or 0*10*10*1(0|1)*.
- Write a regular expression to describe inputs over the alphabet{a, b, c} that are in sorted order.Answer: a*b*c*.
- Write a regular expression for each of the following sets of binary strings. Use only the basic operations.
- contains at least three consecutive 1s
- contains the substring 110
- contains the substring 1101100
- doesn't contain the substring 110
Answers: (0|1)*111(0|1)*, (0|1)*110(0|1)*,(0|1)*1101100(0|1)*, (0|10)*1*.The last one is by far the trickiest.
- Write a regular expression for binary stringswith at least two 0s but not consecutive 0s.
- Write a regular expression for each of the following sets of binary strings. Use only the basic operations.
- has at least 3 characters, and the third character is 0
- number of 0s is a multiple of 3
- starts and ends with the same character
- odd length
- starts with 0 and has odd length, or starts with 1and has even length
- length is at least 1 and at most 3
Answers:(0|1)(0|1)0(0|1)*, 1* | (1*01*01*01*)*, 1(0|1)*1 | 0(0|1)*0 | 0 | 1, (0|1)((0|1)(0|1))*, 0((0|1)(0|1))* | 1(0|1)((0|1)(0|1))*, (0|1) | (0|1)(0|1) | (0|1)(0|1)(0|1).
- For each of the following, indicate how many bit strings oflength exactly 1000 are matched by the regular expression:0(0 | 1)*1, 0*101*, (1 | 01)*.
- Write a regular expression that matches all strings over thealphabet {a, b, c} that contain:
- starts and ends with a
- at most one a
- at least two a's
- an even number of a's
- number of a's plus number of b's is even
- Find long words whose letters are in alphabetical order, e.g., almost and beefily.Answer: use the regular expression'^a*b*c*d*e*f*g*h*i*j*k*l*m*n*o*p*q*r*s*t*u*v*w*x*y*z*$'.
- Write a Java regular expression to match phone numbers,with or without area codes. The area codes should be of the form (609) 555-1234 or 555-1234.
- Find all English words that end with nym.
- Final all English words that contain the trigraph bze.Answer: subzero.
- Find all English words that start with g, contain the trigraphpev and end with e.Answer: grapevine.
- Find all English words that contain the trigraph spband have at least two r's.
- Find the longest English word that can be written with the top row of a standard keyboard.Answer: proprietorier.
- Find all words that contain the four letters a, s, d, and f,not necessarily in that order.Solution: cat words.txt | grep a | grep s | grep d | grep f.
- Given a string of A, C, T, and G, and X, finda string where X matches any single character, e.g.,CATGG is contained in ACTGGGXXAXGGTTT.
- Write a Java regular expression, for use with Validate.java, that validates Social Securitynumbers of the form 123-45-6789.Hint: use d to represent any digit.Answer:[0-9]{3}-[0-9]{2}-[0-9]{4}.
- Modify the previous exercise to make the - optional,so that 123456789 is considered a legal input.
- Write a Java regular expression to match all strings that containexactly five vowels and the vowels are in alphabetical order.Answer:[^aeiou]*a[^aeiou]*e[^aeiou]*i[^aeiou]*o[^aeiou]*u[^aeiou]*
- Write a Java regular expression to match valid Windows XP file names.Such a file name consists of any sequence of characters otherthan
Additionally, it cannot begin with a space or period.
- Write a Java regular expression to match valid OS X file names.Such a file name consists of any sequence of characters other thana colon.Additionally, it cannot begin with a period.
- Given a string s that represents the name of an IP address in dotted quad notation, break it up into its constituent pieces, e.g., 255.125.33.222.Make sure that the four fields are numeric.
- Write a Java regular expression to describe all dates ofthe form Month DD, YYYY where Month consistsof any string of upper or lower case letters, the date is 1 or 2digits, and the year is exactly 4 digits. The comma and spacesare required.
- Write a Java regular expression to describe valid IP addressesof the form a.b.c.d where each letter can represent 1, 2, or 3digits, and the periods are required. Yes: 196.26.155.241.
- Write a Java regular expression to match license platesthat start with 4 digits and end with two uppercase letters.
- Write a regular expression to extract the coding sequencefrom a DNA string. It starts with the ATG codon and ends with a stop codon (TAA, TAG, or TGA).reference
- Write a regular expression to check for the sequencerGATCy: that is, does it start with A or G, then GATC, and thenT or C.
- Write a regular expression to check whether a sequence containstwo or more repeats of the the GATA tetranucleotide.
- Modify Validate.javato make the searches case insensitive.Hint: use the (?i) embedded flag.
- Write a Java regular expression to match various spellingsof Libyan dictator Moammar Gadhafi's last name usingthe folling template: (i) starts with K, G, Q, (ii) optionallyfollowed by H, (iii) followed by AD, (iv) optionally followedby D, (v) optionally followed by H, (vi) optionally followedby AF, (vii) optionally followed by F, (vii) ends with I.
- Write a Java program that reads in an expressionlike (K|G|Q)[H]AD[D][H]AF[F]I and prints out allmatching strings. Here the notation [x] means0 or 1 copy of the letter x.
- Why doesn't s.replaceAll('A', 'B'); replace alloccurrences of the letter A with B in the string s?
Answer: Use s = s.replaceAll('A', 'B'); insteadThe method replaceAll returns the resulting string, butdoes not change s itself.Strings are immutable.
- Write a program Clean.javathat reads in text from standard input andprints it back out, removing any trailing whitespace on a lineand replacing all tabs with 4 spaces.
Hint: use replaceAll() and the regular expressions for whitespace.
- Write a regular expression to match all of the textbetween the text a href =' and the next '.Answer: href='(.*?)'. The? makes the .* reluctant instead of greedy.In Java, use Pattern.compile('href='(.*?)', Pattern.CASE_INSENSITIVE)to escape the backslash characters.
- Use regular expressions to extract all of the text between the tags</tt> and <tt><title></tt>.The <tt>(?i)</tt> is another way to make the match case insensitive.The <tt>$2</tt> refers to the second captured subsequence, i.e.,the stuff between the <tt>title</tt> tags.</li><li>Write a regular expression to match all of the textbetween <TD ..> and </TD> tags.<em>Answer</em>: <tt> <TD[^>]*>([^<]*)</TD> </tt></li></ol><h4>Creative Exercises</h4><ol><li><b>FMR-1 triplet repeat region.</b>'The human FMR-1 gene sequence contains a triplet repeat regionin which the sequence CGG or AGG is repeated a number of times. The numberof triplets is highly variable between individuals, and increasedcopy number is associated with fragile X syndrome, a genetic diseasethat causes mental retardation and other symptoms in one out of2000 children.' (Reference: Biological Sequence Analysis byDurbin et al). The pattern is bracket by GCG and CTG,so we get the regular expression GCG (CGG | AGG)* CTG.</li><li><b>Ad blocking.</b>Adblock uses regularexpressions to block banner adds under the Mozilla and Firebirdbrowsers.</li><li><b>Parsing text files.</b>A more advanced example where we want to extract specific pieces of thematching input. This program typifies the process of parsing scientific input data.</li><li><b>PROSITE to Java regular expression.</b>Write a program to read in a PROSITE pattern and print outthe corresponding Java regular expression.PROSITE is the 'first and most famous' database of protein families and domains.Its main use it to determine the function of uncharacterized proteins translated from genomic sequences. Biologists usePROSITEpattern syntax rules to search for patterns in biological data.Here is the raw data for CBD FUNGAL(accession code PS00562). Each line contains various information. Perhapsthe most interesting line is the one that begins with PA - it containsthe pattern that describes the protein motif.Such patterns are useful because they often correspond to functional or structural features.<p>Each uppercase letter corresponds to one amino acid residue.The alphabet consists of uppercase letters corresponding to the 2x amino acids.The <tt>-</tt> character means concatenation.For example, the pattern above begins with CGG (Cys-Gly-Gly).The notation <tt>x</tt> plays the role of a wildcard - it matches any amino acid. This corresponds to <tt>.</tt> in our notation.Parentheses are used to specify repeats:<tt>x(2)</tt> meansexactly two amino acids, and <tt>x(4,7)</tt> means between 4 and 7 aminoacids. This corresponds to <tt>.{2}</tt> and <tt>.{4,7}</tt> in Javanotation.Curly braces are used to specify forbidden residues:{CG} means any residue other than C or G.The asterisk has its usual meaning.</p></li><li><b>Text to speech synthesis.</b>Original motivation for grep. 'For example, how do you cope with the digraph ui, which is pronounced many different ways: fruit, guile, guilty, anguish, intuit, beguine?'</li><li><b>Challenging regular expressions.</b>Write a regular expression for each of the following sets of binary strings. Use only the basic operations.<ol><li>any string except 11 or 111</li><li>every odd symbol is a 1</li><li>contains at least two 0s and at most one 1</li><li>no consecutive 1s</li></ol></li><li><b>Binary divisibility.</b>Write a regular expression for each of the following sets of binary strings. Use only the basic operations.<ol><li>bit string interpreted as binary number is divisible by 3</li><li>bit string interpreted as binary number is divisible by 123</li></ol></li><li><b>Boston accent.</b>Write a program to replace all of the r's with h's to translatea sentence like 'Park the car in Harvard yard' into the Bostonianversion 'Pahk the cah in Hahvahd yahd'.</li><li><b>File extension.</b>Write a program that takes the name of a file as a command line argumentand prints out its file type extension. The <em>extension</em>is the sequence of characters following the last <tt>.</tt>.For example the file <tt>sun.gif</tt> has the extension <tt>gif</tt>.Hint: use <tt>split('.')</tt>; recall that <tt>.</tt> is a regular expression meta-character, so you need to escape it.</li><li><b>Reverse subdomains.</b>For web log analysis, it is convenient to organize web trafficbased on subdomains like <tt>wayne.faculty.cs.princeton.edu</tt>.Write a program to read in a domain name and print it outin reverse order like<tt>edu.princeton.cs.faculty.wayne</tt>.</li><li><b>Bank robbery.</b>You just witnessed a bank robbery and got a partial license plateof the getaway vehicle. It started with <tt>ZD</tt>, hada <tt>3</tt> somewhere in the middle and ended with <tt>V</tt>.Help the police officer write regular expression for this plate.</li><li><b>Regular expression for permutations.</b>Find the shortest regular expression (using only the basic operations)you can for the set of all permutations on N elements for N = 5 or 10.For example if N = 3, then the language is abc, acb, bac,bca, cab, cba.<em>Answer</em>: difficult. Solution has length exponentialin N.</li><li><b>Parsing quoted strings.</b>Read in a text file and print out all quote strings.Use a regular expression like <tt>'[^']*'</tt>, but needto worry about escaping the quotation marks.</li><li><b>Parsing HTML.</b>A >, optionally followed by whitespace, followed by <tt>a</tt>,followed by whitespace, followed by <tt>href</tt>, optionallyfollowed by whitespace, followed by <tt>=</tt>, optionally followed by whitespace, followed by <tt>'http://</tt>,followed by characters until <tt>'</tt>, optionally followed bywhitespace, then a <tt><</tt>.</li><li><b>Subsequence.</b>Given a string s, determine whether it is a subsequence of anotherstring t. For example, abc is a subsequence of achfdbaabgabcaabg.Use a regular expression. Now repeat the process without usingregular expressions.Answer: (a) a.*b.*c.*, (b) use a greedy algorithm.</li><li><b>Huntington's disease diagnostic.</b>The gene that causes Huntington's disease is located on chromosome 4,and has a variable number of repeats of the CAG trinucleotide repeat.Write a program to determine the numberof repeats and print <tt>will not develop HD</tt> If the number of repeatsis less than 26, <tt>offspring at risk</tt> if the number is 37-35,<tt>at risk</tt> if the number is between 36 and 39, and <tt>will develop HD</tt>if the number is greater than or equal to 40.This is how Huntington's disease is identified in genetic testing.</li><li><b>Gene finder.</b>A gene is a substring of a genome that starts withthe start codon (ATG), end with a stop codon (TAG, TAA, TAG, or TGA)and consists of a sequence of codons (nucleotide triplets)other than the start or stop codons. The gene is thesubstring in between the start and stop codons.</li><li><b>Repeat finder.</b>Write a program <tt>Repeat.java</tt> that takes two command line arguments,and finds the maximum number of repeats of the first command line argumentin the file specified by the second command line argument.</li><li><b>Character filter.</b>Given a string <tt>t</tt> of<em>bad characters</em>, e.g. <tt>t = '!@#$%^&*()-_=+'</tt>,write a function to read in another string <tt>s</tt> andreturn the result of removing all of the bad characters.</li><li><b>Wildcard pattern matcher.</b>Without using Java's built in regular expressions,write a program Wildcard.javato find all words in the dictionary matching a givenpattern. The special symbol * matches any zero or more characters.So, for example the pattern 'w*ard' matches the word 'ward' and'wildcard'. The special symbol . matches any one character.Your program should read the pattern as a command line parameterand the list of words (separated by whitespace) from standard input.</li><li><b>Wildcard pattern matcher.</b>Repeat the previous exercise, but this time use Java'sbuilt in regular expressions. <em>Warning:</em> in the contextof wildcards, * has a different meaning than with regular expressions.</li><li><b>Search and replace.</b>Word processors allow you to search for all occurrences ofa given query string and replace each with another replacement string.Write a programSearchAndReplace.java thattakes two strings as command line inputs,reads in data from standard input, and replaces all occurrences ofthe first string with the second string, andsends the results to standard output.<em>Hint</em>: use the method <tt>String.replaceAll</tt>.</li><li><b>Password validator.</b>Suppose that for security reasons you require all passwordsto have at least one of the following charactersWrite a regular expression for use with <tt>String.matches</tt>that returns <tt>true</tt> if and only if the password contains one of therequired characters.<em>Answer</em>: '^[^~!@#$%^&*|]+$' </li><li><b>Alphanumeric filter.</b>Write a program Filter.javato read in text from standard input and eliminate allcharacters that are not whitespace or alpha-numeric.<em>Answer</em> here's the key line.</li><li><b>Converting tabs to spaces.</b>Write a program to convert all tabs in a Java source file to 4 spaces.</li><li><b>Parsing delimited text files.</b>A popular way to store a database is in a text file with one record per line,and each field separated by a special character called the delimiter.Write a program Tokenizer.java that reads in two command line parameters, a delimiter character andthe name of the file, and creates an array of tokens.</li><li><b>Parsing delimited text files.</b>Repeat the previous exercise, but use the <tt>String</tt> librarymethod <tt>split()</tt>.</li><li><b>Checking a file format.</b></li><li><b>Misspellings.</b>Write a Java program to verify that this list ofcommon misspellingsadapted from Wikipediacontains only lines of the form<p>where the first word is the misspelling and the string in parentheses isa possible replacement.</p></li><li><b>Size of DFA is exponential in size of RE.</b>Give a RE for the set of all bitstrings whose kth to the last character equals 1.The size of the RE should be linear in k.Now, give a DFA for the same set of bitstrings.How many states does it use?<p><em>Hint</em>: every DFA for this set of bitstrings must have at least2^k states.</p></li></ol><br><p><em>Last modified on August 27, 2016.</em><br>Copyright © 2000–2019Robert SedgewickandKevin Wayne.All rights reserved.</p><p>An introduction to writing mathematical expressions in Matplotlib.</p><p>You can use a subset TeX markup in any matplotlib text string by placing itinside a pair of dollar signs ($).</p><p>Note that you do not need to have TeX installed, since Matplotlib shipsits own TeX expression parser, layout engine, and fonts. The layout engineis a fairly direct adaptation of the layout algorithms in Donald Knuth'sTeX, so the quality is quite good (matplotlib also provides a <code><span>usetex</span></code>option for those who do want to call out to TeX to generate their text (see<span>Text rendering With LaTeX</span>).</p><h3 id='1-31-31-3-equals'>1 3+1 3+1 3 Equals</h3><p>Any text element can use math text. You should use raw strings (precede thequotes with an <code><span>'r'</span></code>), and surround the math text with dollar signs ($), asin TeX. Regular text and mathtext can be interleaved within the same string.Mathtext can use DejaVu Sans (default), DejaVu Serif, the Computer Modern fonts(from (La)TeX), STIX fonts (with are designedto blend well with Times), or a Unicode font that you provide. The mathtextfont can be selected with the customization variable <code><span>mathtext.fontset</span></code> (see<span>Customizing Matplotlib with style sheets and rcParams</span>)</p><p>Here is a simple example:</p><p>produces 'alpha > beta'.</p><p>Whereas this:</p><p>produces '.</p><p>Note</p><p>Mathtext should be placed between a pair of dollar signs ($). To make iteasy to display monetary values, e.g., '$100.00', if a single dollar signis present in the entire string, it will be displayed verbatim as a dollarsign. This is a small change from regular TeX, where the dollar sign innon-math text would have to be escaped ('$').</p><p>Note</p><p>While the syntax inside the pair of dollar signs ($) aims to be TeX-like,the text outside does not. In particular, characters such as:</p><p>have special meaning outside of math mode in TeX. Therefore, thesecharacters will behave differently depending on the rcParam <code><span>text.usetex</span></code>flag. See the <span>usetex tutorial</span> for moreinformation.</p><h2>Subscripts and superscripts¶</h2><p>To make subscripts and superscripts, use the <code><span>'_'</span></code> and <code><span>'^'</span></code> symbols:</p><div>[alpha_i > beta_i]</div><p>Some symbols automatically put their sub/superscripts under and over theoperator. For example, to write the sum of from to, you could do:</p><div>[sum_{i=0}^infty x_i]</div><h2>Fractions, binomials, and stacked numbers¶</h2><p>Fractions, binomials, and stacked numbers can be created with the<code><span>frac{}{}</span></code>, <code><span>binom{}{}</span></code> and <code><span>genfrac{}{}{}{}{}{}</span></code> commands,respectively:</p><p><a href='https://policesoft.mystrikingly.com/blog/mixed-in-key-ru' title='Mixed in key ru'>Mixed in key ru</a>. produces</p><div>[frac{3}{4} binom{3}{4} stackrel{}{}{0}{}{3}{4}]</div><p>Fractions can be arbitrarily nested:</p><h3 id='expressions-1-3-1-x-2'>Expressions 1 3 1 X 2</h3><p>produces</p><div>[frac{5 - frac{1}{x}}{4}]</div><p>Note that special care needs to be taken to place parentheses and bracketsaround fractions. Doing things the obvious way produces brackets that are toosmall:</p><div>[(frac{5 - frac{1}{x}}{4})]</div><p>The solution is to precede the bracket with <code><span>left</span></code> and <code><span>right</span></code> to informthe parser that those brackets encompass the entire object.:</p><div>[left(frac{5 - frac{1}{x}}{4}right)]</div><h2>Radicals¶</h2><p>Radicals can be produced with the <code><span>sqrt[]{}</span></code> command. For example:</p><div>[sqrt{2}]</div><p>Any base can (optionally) be provided inside square brackets. Note that thebase must be a simple expression, and can not contain layout commands such asfractions or sub/superscripts:</p><div>[sqrt[3]{x}]</div><h2>Fonts¶</h2><p>The default font is <em>italics</em> for mathematical symbols.</p><p>Note</p><p>This default can be changed using the <code><span>mathtext.default</span></code> rcParam. This isuseful, for example, to use the same font as regular non-math text for mathtext, by setting it to <code><span>regular</span></code>.</p><p><a href='https://policesoft.mystrikingly.com/blog/money-pro-2-0-manage-money-like-a-programmer' title='Money pro 2 0 – manage money like a programmer'>Money pro 2 0 – manage money like a programmer</a>. To change fonts, e.g., to write 'sin' in a Roman font, enclose the text in afont command:</p><div>[s(t) = mathcal{A}mathrm{sin}(2 omega t)]</div><p>More conveniently, many commonly used function names that are typeset ina Roman font have shortcuts. So the expression above could be written asfollows:</p><div>[s(t) = mathcal{A}sin(2 omega t)]</div><p>Here 's' and 't' are variable in italics font (default), 'sin' is in Romanfont, and the amplitude 'A' is in calligraphy font. Note in the example abovethe calligraphy <code><span>A</span></code> is squished into the <code><span>sin</span></code>. You can use a spacingcommand to add a little whitespace between them:</p><div>[s(t) = mathcal{A},sin(2 omega t)]</div><p>The choices available with all fonts are:</p><table border='1'><thead valign='bottom'><tr><th>Command</th><th>Result</th></tr></thead><tbody valign='top'><tr><td><code><span>mathrm{Roman}</span></code></td></tr><tr><td><code><span>mathit{Italic}</span></code></td></tr><tr><td><code><span>mathtt{Typewriter}</span></code></td></tr><tr><td><code><span>mathcal{CALLIGRAPHY}</span></code></td></tr></tbody></table><p>When using the STIX fonts, you also have thechoice of:</p><table border='1'><thead valign='bottom'><tr><th>Command</th><th>Result</th></tr></thead><tbody valign='top'><tr><td><code><span>mathbb{blackboard}</span></code></td></tr><tr><td><code><span>mathrm{mathbb{blackboard}}</span></code></td></tr><tr><td><code><span>mathfrak{Fraktur}</span></code></td></tr><tr><td><code><span>mathsf{sansserif}</span></code></td></tr><tr><td><code><span>mathrm{mathsf{sansserif}}</span></code></td></tr></tbody></table><p>There are also three global 'font sets' to choose from, which areselected using the <code><span>mathtext.fontset</span></code> parameter in <span>matplotlibrc</span>.</p><p><code><span>cm</span></code>: <strong>Computer Modern (TeX)</strong></p><p><code><span>stix</span></code>: <strong>STIX</strong> (designed to blend well with Times)</p><p><code><span>stixsans</span></code>: <strong>STIX sans-serif</strong></p><p>Additionally, you can use <code><span>mathdefault{..}</span></code> or its alias<code><span>mathregular{..}</span></code> to use the font used for regular text outside ofmathtext. There are a number of limitations to this approach, most notablythat far fewer symbols will be available, but it can be useful to make mathexpressions blend well with other text in the plot.</p><h3>Custom fonts¶</h3><p>mathtext also provides a way to use custom fonts for math. This method isfairly tricky to use, and should be considered an experimental feature forpatient users only. By setting the rcParam <code><span>mathtext.fontset</span></code> to <code><span>custom</span></code>,you can then set the following parameters, which control which font file to usefor a particular set of math characters.</p><table border='1'><thead valign='bottom'><tr><th>Parameter</th><th>Corresponds to</th></tr></thead><tbody valign='top'><tr><td><code><span>mathtext.it</span></code></td><td><code><span>mathit{}</span></code> or default italic</td></tr><tr><td><code><span>mathtext.rm</span></code></td><td><code><span>mathrm{}</span></code> Roman (upright)</td></tr><tr><td><code><span>mathtext.tt</span></code></td><td><code><span>mathtt{}</span></code> Typewriter (monospace)</td></tr><tr><td><code><span>mathtext.bf</span></code></td><td><code><span>mathbf{}</span></code> bold italic</td></tr><tr><td><code><span>mathtext.cal</span></code></td><td><code><span>mathcal{}</span></code> calligraphic</td></tr><tr><td><code><span>mathtext.sf</span></code></td><td><code><span>mathsf{}</span></code> sans-serif</td></tr></tbody></table><p>Each parameter should be set to a fontconfig font descriptor (as defined in theyet-to-be-written font chapter).</p><p>The fonts used should have a Unicode mapping in order to find anynon-Latin characters, such as Greek. If you want to use a math symbolthat is not contained in your custom fonts, you can set the rcParam<code><span>mathtext.fallback_to_cm</span></code> to <code><span>True</span></code> which will cause the mathtext systemto use characters from the default Computer Modern fonts whenever a particularcharacter can not be found in the custom font.</p><p>Note that the math glyphs specified in Unicode have evolved over time, and manyfonts may not have glyphs in the correct place for mathtext.</p><h2>Accents¶</h2><p>An accent command may precede any symbol to add an accent above it. There arelong and short forms for some of them.</p><table border='1'><thead valign='bottom'><tr><th>Command</th><th>Result</th></tr></thead><tbody valign='top'><tr><td><code><span>acute</span><span>a</span></code> or <code><span>'a</span></code></td></tr><tr><td><code><span>bar</span><span>a</span></code></td></tr><tr><td><code><span>breve</span><span>a</span></code></td></tr><tr><td><code><span>ddot</span><span>a</span></code> or <code><span>'a</span></code></td></tr><tr><td><code><span>dot</span><span>a</span></code> or <code><span>.a</span></code></td></tr><tr><td><code><span>grave</span><span>a</span></code> or <code><span>`a</span></code></td></tr><tr><td><code><span>hat</span><span>a</span></code> or <code><span>^a</span></code></td></tr><tr><td><code><span>tilde</span><span>a</span></code> or <code><span>~a</span></code></td></tr><tr><td><code><span>vec</span><span>a</span></code></td></tr><tr><td><code><span>overline{abc}</span></code></td></tr></tbody></table><p>In addition, there are two special accents that automatically adjust to thewidth of the symbols below:</p><p>Care should be taken when putting accents on lower-case i's and j's. Note thatin the following <code><span>imath</span></code> is used to avoid the extra dot over the i:</p><div>[hat i hat imath]</div><h2>Symbols¶</h2><p>You can also use a large number of the TeX symbols, as in <code><span>infty</span></code>,<code><span>leftarrow</span></code>, <code><span>sum</span></code>, <code><span>int</span></code>.</p><p><strong>Lower-case Greek</strong></p>
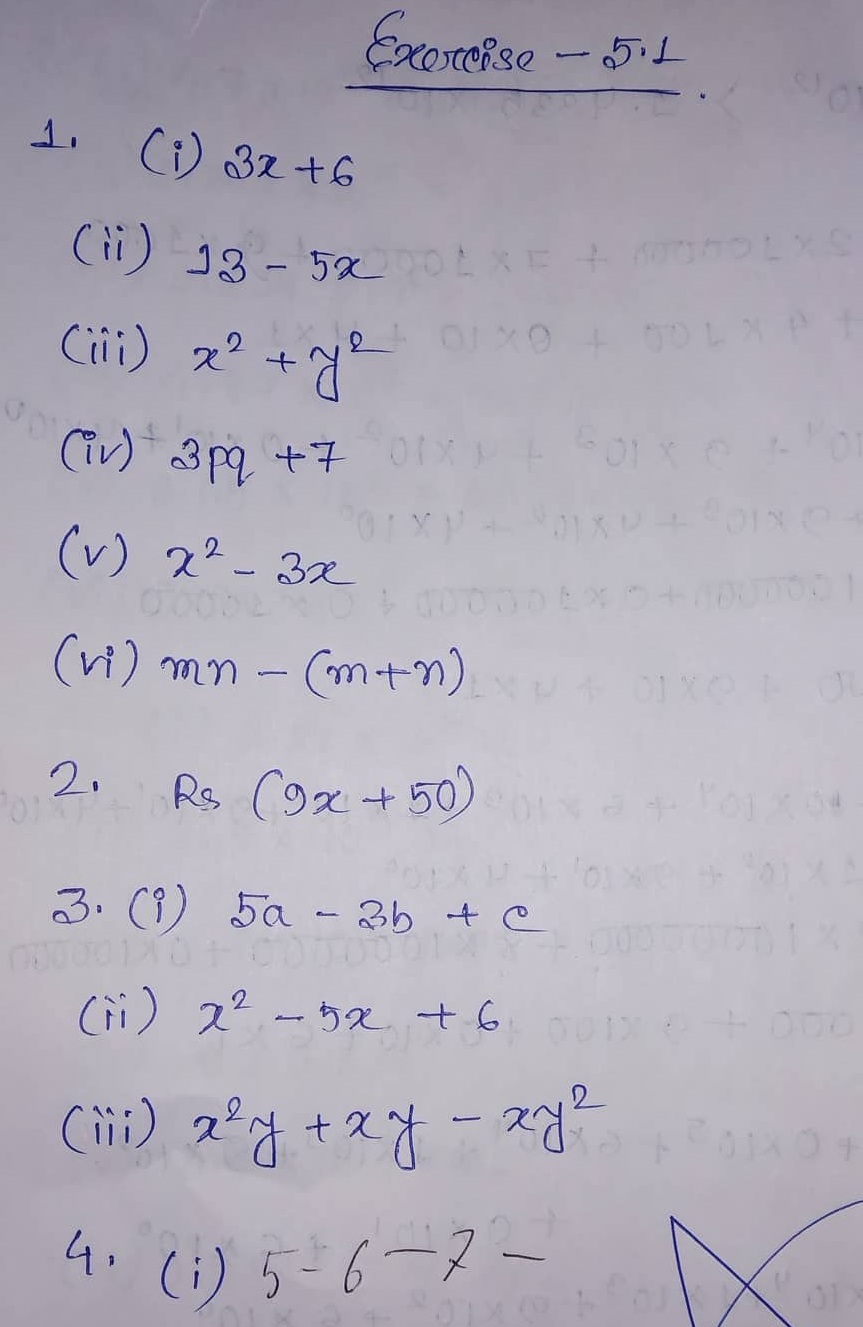
α alpha | β beta | χ chi | δ delta | ϝ digamma | ε epsilon |
η eta | γ gamma | ι iota | κ kappa | λ lambda | μ mu |
ν nu | ω omega | ϕ phi | π pi | ψ psi | ρ rho |
σ sigma | τ tau | θ theta | υ upsilon | ε varepsilon | ϰ varkappa |
φ varphi | ϖ varpi | ϱ varrho | ς varsigma | ϑ vartheta | ξ xi |
ζ zeta |
Upper-case Greek
Δ Delta | Γ Gamma | Λ Lambda | Ω Omega | Φ Phi | Π Pi | Ψ Psi | Σ Sigma |
Θ Theta | Υ Upsilon | Ξ Xi | ℧ mho | ∇ nabla |
Hebrew
Delimiters
3.1 Simplify Rational Expressions
/ / | [ [ | ⇓ Downarrow | ⇑ Uparrow | ‖ Vert | backslash |
↓ downarrow | ⟨ langle | ⌈ lceil | ⌊ lfloor | ⌞ llcorner | ⌟ lrcorner |
⟩ rangle | ⌉ rceil | ⌋ rfloor | ⌜ ulcorner | ↑ uparrow | ⌝ urcorner |
{ { | } } | ] ] |
Big symbols
⋂ bigcap | ⋃ bigcup | ⨀ bigodot | ⨁ bigoplus | ⨂ bigotimes | ⨄ biguplus |
⋁ bigvee | ⋀ bigwedge | ∐ coprod | ∫ int | ∮ oint | ∏ prod |
∑ sum |
Standard function names
Pr Pr | arccos arccos | arcsin arcsin | arctan arctan | arg arg | cos cos |
cosh cosh | cot cot | coth coth | csc csc | deg deg | det det |
dim dim | exp exp | gcd gcd | hom hom | inf inf | ker ker |
lg lg | lim lim | liminf liminf | limsup limsup | ln ln | log log |
max max | min min | sec sec | sin sin | sinh sinh | sup sup |
tan tan | tanh tanh |
Binary operation and relation symbols
≎ Bumpeq | ⋒ Cap | ⋓ Cup | ≑ Doteq |
⨝ Join | ⋐ Subset | ⋑ Supset | ⊩ Vdash |
⊪ Vvdash | ≈ approx | ≊ approxeq | ∗ ast |
≍ asymp | ϶ backepsilon | ∽ backsim | ⋍ backsimeq |
⊼ barwedge | ∵ because | ≬ between | ○ bigcirc |
▽ bigtriangledown | △ bigtriangleup | ◀ blacktriangleleft | ▶ blacktriangleright |
⊥ bot | ⋈ bowtie | ⊡ boxdot | ⊟ boxminus |
⊞ boxplus | ⊠ boxtimes | ∙ bullet | ≏ bumpeq |
∩ cap | ⋅ cdot | ∘ circ | ≗ circeq |
≔ coloneq | ≅ cong | ∪ cup | ⋞ curlyeqprec |
⋟ curlyeqsucc | ⋎ curlyvee | ⋏ curlywedge | † dag |
⊣ dashv | ‡ ddag | ⋄ diamond | ÷ div |
⋇ divideontimes | ≐ doteq | ≑ doteqdot | ∔ dotplus |
⌆ doublebarwedge | ≖ eqcirc | ≕ eqcolon | ≂ eqsim |
⪖ eqslantgtr | ⪕ eqslantless | ≡ equiv | ≒ fallingdotseq |
⌢ frown | ≥ geq | ≧ geqq | ⩾ geqslant |
≫ gg | ⋙ ggg | ⪺ gnapprox | ≩ gneqq |
⋧ gnsim | ⪆ gtrapprox | ⋗ gtrdot | ⋛ gtreqless |
⪌ gtreqqless | ≷ gtrless | ≳ gtrsim | ∈ in |
⊺ intercal | ⋋ leftthreetimes | ≤ leq | ≦ leqq |
⩽ leqslant | ⪅ lessapprox | ⋖ lessdot | ⋚ lesseqgtr |
⪋ lesseqqgtr | ≶ lessgtr | ≲ lesssim | ≪ ll |
⋘ lll | ⪹ lnapprox | ≨ lneqq | ⋦ lnsim |
⋉ ltimes | ∣ mid | ⊧ models | ∓ mp |
⊯ nVDash | ⊮ nVdash | ≉ napprox | ≇ ncong |
≠ ne | ≠ neq | ≠ neq | ≢ nequiv |
≱ ngeq | ≯ ngtr | ∋ ni | ≰ nleq |
≮ nless | ∤ nmid | ∉ notin | ∦ nparallel |
⊀ nprec | ≁ nsim | ⊄ nsubset | ⊈ nsubseteq |
⊁ nsucc | ⊅ nsupset | ⊉ nsupseteq | ⋪ ntriangleleft |
⋬ ntrianglelefteq | ⋫ ntriangleright | ⋭ ntrianglerighteq | ⊭ nvDash |
⊬ nvdash | ⊙ odot | ⊖ ominus | ⊕ oplus |
⊘ oslash | ⊗ otimes | ∥ parallel | ⟂ perp |
⋔ pitchfork | ± pm | ≺ prec | ⪷ precapprox |
≼ preccurlyeq | ≼ preceq | ⪹ precnapprox | ⋨ precnsim |
≾ precsim | ∝ propto | ⋌ rightthreetimes | ≓ risingdotseq |
⋊ rtimes | ∼ sim | ≃ simeq | ∕ slash |
⌣ smile | ⊓ sqcap | ⊔ sqcup | ⊏ sqsubset |
⊏ sqsubset | ⊑ sqsubseteq | ⊐ sqsupset | ⊐ sqsupset |
⊒ sqsupseteq | ⋆ star | ⊂ subset | ⊆ subseteq |
⫅ subseteqq | ⊊ subsetneq | ⫋ subsetneqq | ≻ succ |
⪸ succapprox | ≽ succcurlyeq | ≽ succeq | ⪺ succnapprox |
⋩ succnsim | ≿ succsim | ⊃ supset | ⊇ supseteq |
⫆ supseteqq | ⊋ supsetneq | ⫌ supsetneqq | ∴ therefore |
× times | ⊤ top | ◁ triangleleft | ⊴ trianglelefteq |
≜ triangleq | ▷ triangleright | ⊵ trianglerighteq | ⊎ uplus |
⊨ vDash | ∝ varpropto | ⊲ vartriangleleft | ⊳ vartriangleright |
⊢ vdash | ∨ vee | ⊻ veebar | ∧ wedge |
≀ wr |
Arrow symbols
⇓ Downarrow | ⇐ Leftarrow | ⇔ Leftrightarrow | ⇚ Lleftarrow |
⟸ Longleftarrow | ⟺ Longleftrightarrow | ⟹ Longrightarrow | ↰ Lsh |
⇗ Nearrow | ⇖ Nwarrow | ⇒ Rightarrow | ⇛ Rrightarrow |
↱ Rsh | ⇘ Searrow | ⇙ Swarrow | ⇑ Uparrow |
⇕ Updownarrow | ↺ circlearrowleft | ↻ circlearrowright | ↶ curvearrowleft |
↷ curvearrowright | ⤎ dashleftarrow | ⤏ dashrightarrow | ↓ downarrow |
⇊ downdownarrows | ⇃ downharpoonleft | ⇂ downharpoonright | ↩ hookleftarrow |
↪ hookrightarrow | ⇝ leadsto | ← leftarrow | ↢ leftarrowtail |
↽ leftharpoondown | ↼ leftharpoonup | ⇇ leftleftarrows | ↔ leftrightarrow |
⇆ leftrightarrows | ⇋ leftrightharpoons | ↭ leftrightsquigarrow | ↜ leftsquigarrow |
⟵ longleftarrow | ⟷ longleftrightarrow | ⟼ longmapsto | ⟶ longrightarrow |
↫ looparrowleft | ↬ looparrowright | ↦ mapsto | ⊸ multimap |
⇍ nLeftarrow | ⇎ nLeftrightarrow | ⇏ nRightarrow | ↗ nearrow |
↚ nleftarrow | ↮ nleftrightarrow | ↛ nrightarrow | ↖ nwarrow |
→ rightarrow | ↣ rightarrowtail | ⇁ rightharpoondown | ⇀ rightharpoonup |
⇄ rightleftarrows | ⇄ rightleftarrows | ⇌ rightleftharpoons | ⇌ rightleftharpoons |
⇉ rightrightarrows | ⇉ rightrightarrows | ↝ rightsquigarrow | ↘ searrow |
↙ swarrow | → to | ↞ twoheadleftarrow | ↠ twoheadrightarrow |
↑ uparrow | ↕ updownarrow | ↕ updownarrow | ↿ upharpoonleft |
↾ upharpoonright | ⇈ upuparrows |
Miscellaneous symbols
$ $ | Å AA | Ⅎ Finv | ⅁ Game |
ℑ Im | ¶ P | ℜ Re | § S |
∠ angle | ‵ backprime | ★ bigstar | ■ blacksquare |
▴ blacktriangle | ▾ blacktriangledown | ⋯ cdots | ✓ checkmark |
® circledR | Ⓢ circledS | ♣ clubsuit | ∁ complement |
© copyright | ⋱ ddots | ♢ diamondsuit | ℓ ell |
∅ emptyset | ð eth | ∃ exists | ♭ flat |
∀ forall | ħ hbar | ♡ heartsuit | ℏ hslash |
∭ iiint | ∬ iint | ı imath | ∞ infty |
ȷ jmath | … ldots | ∡ measuredangle | ♮ natural |
¬ neg | ∄ nexists | ∰ oiiint | ∂ partial |
′ prime | ♯ sharp | ♠ spadesuit | ∢ sphericalangle |
ß ss | ▿ triangledown | ∅ varnothing | ▵ vartriangle |
⋮ vdots | ℘ wp | ¥ yen |
If a particular symbol does not have a name (as is true of many of the moreobscure symbols in the STIX fonts), Unicode characters can also be used:
Example¶
Here is an example illustrating many of these features in context.
Keywords: matplotlib code example, codex, python plot, pyplotGallery generated by Sphinx-Gallery